- The Dev Loop
- Posts
- The Golang Chronicle #18 – Building Scalable Microservices in Go: A Practical Guide
The Golang Chronicle #18 – Building Scalable Microservices in Go: A Practical Guide
Everything You Need to Know About Microservices in Go
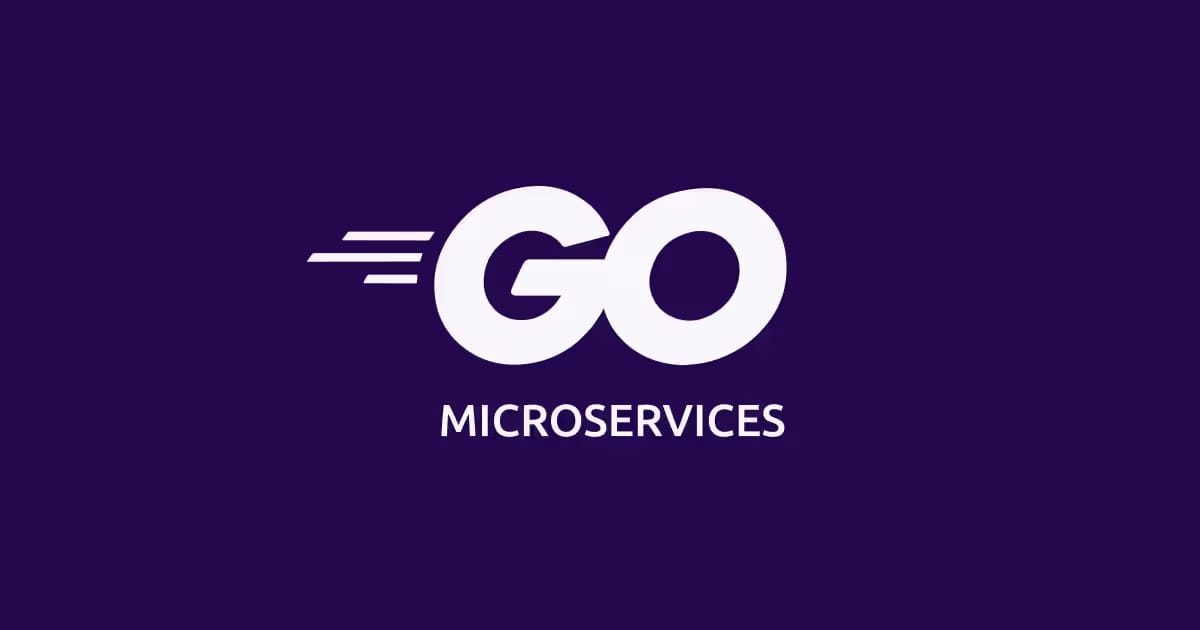
📢 Introduction: Why Go for Microservices?
Microservices architecture has become the go-to solution for building scalable, maintainable, and resilient applications. Go, with its lightweight concurrency model, efficient memory management, and high performance, is an excellent choice for developing microservices.
In this edition of The Golang Chronicle, we’ll explore how to design, build, and scale microservices using Go, covering essential concepts, tools, and best practices.
🛠️ 1. Key Principles of Microservices Architecture
Before diving into implementation, let’s understand the fundamental principles that define a well-structured microservices system:
Single Responsibility: Each microservice should have a well-defined purpose and handle a specific function.
Independence: Services should be loosely coupled and able to operate independently.
Scalability: Each service should be scalable based on demand.
Resilience: Fault tolerance is critical—services should recover from failures gracefully.
Observability: Logging, monitoring, and tracing are essential for debugging and performance optimization.
⚙️ 2. Setting Up a Go Microservice
A typical Go microservice consists of:
HTTP Server: Using
net/http
or frameworks likeGin
orEcho
.Database Integration: Connecting with SQL (PostgreSQL, MySQL) or NoSQL (MongoDB).
Communication Mechanisms: REST, gRPC, or messaging queues like Kafka.
Configuration Management: Using environment variables or configuration files.
Example: Basic REST API with Gin
package main
import (
"fmt"
"net/http"
"github.com/gin-gonic/gin"
)
func main() {
router := gin.Default()
router.GET("/health", func(c *gin.Context) {
c.JSON(http.StatusOK, gin.H{"status": "healthy"})
})
router.Run(":8080")
}
This sets up a simple microservice with a health check endpoint.
🔗 3. Communication Between Microservices
Microservices communicate using different strategies, including:
REST APIs: Simple and widely used, but may have overhead for high-performance systems.
gRPC: A high-performance RPC framework for faster, efficient communication.
Message Brokers: Kafka, RabbitMQ, or NATS for event-driven communication.
Example: Defining a gRPC Service
syntax = "proto3";
service UserService {
rpc GetUser (UserRequest) returns (UserResponse);
}
message UserRequest {
string id = 1;
}
message UserResponse {
string name = 1;
int32 age = 2;
}
🏗️ 4. Managing State: Database & Caching
To ensure microservices handle data effectively, consider these approaches:
Databases: PostgreSQL (SQL) or MongoDB (NoSQL) based on use cases.
Caching: Redis or Memcached for reducing database load.
Event Sourcing: Storing events instead of direct updates (useful for audits and scalability).
Example: Connecting to PostgreSQL in Go
import (
"database/sql"
_ "github.com/lib/pq"
)
func connectDB() (*sql.DB, error) {
connStr := "user=postgres dbname=mydb sslmode=disable"
return sql.Open("postgres", connStr)
}
📊 5. Observability: Logging, Monitoring, and Tracing
Observability ensures that microservices run smoothly in production. Key practices include:
Logging: Use structured logging libraries like
logrus
orzerolog
.Monitoring: Prometheus + Grafana for real-time metrics.
Distributed Tracing: OpenTelemetry or Jaeger for tracing requests across services.
Example: Logging with Logrus
import (
"github.com/sirupsen/logrus"
)
func main() {
log := logrus.New()
log.Info("Microservice started")
}
🚀 6. Scaling Microservices in Production
Scalability is crucial for handling high traffic. Strategies include:
Containerization: Use Docker to package services for consistent deployment.
Orchestration: Kubernetes (K8s) for managing service deployment and scaling.
Load Balancing: Nginx, HAProxy, or API gateways like Kong for traffic distribution.
CI/CD Pipelines: Automate deployments with GitHub Actions or GitLab CI/CD.
Example: Running a Go Microservice in Docker
Dockerfile:
FROM golang:1.19
WORKDIR /app
COPY . .
RUN go mod tidy
RUN go build -o service
CMD ["/app/service"]
🌟 Conclusion: Build Scalable & Maintainable Go Microservices
Go’s speed, efficiency, and powerful concurrency model make it a top choice for building microservices. By following best practices, leveraging the right tools, and focusing on scalability, you can create robust, high-performance services that power modern applications.
💻 Join the GoLang Community!
Stay tuned for more insights in the next edition of The Golang Chronicle! Have questions or topic ideas? We’d love to hear from you.
Go Community: The Dev Loop Community
Cheers,
The Dev Loop Team 🚀